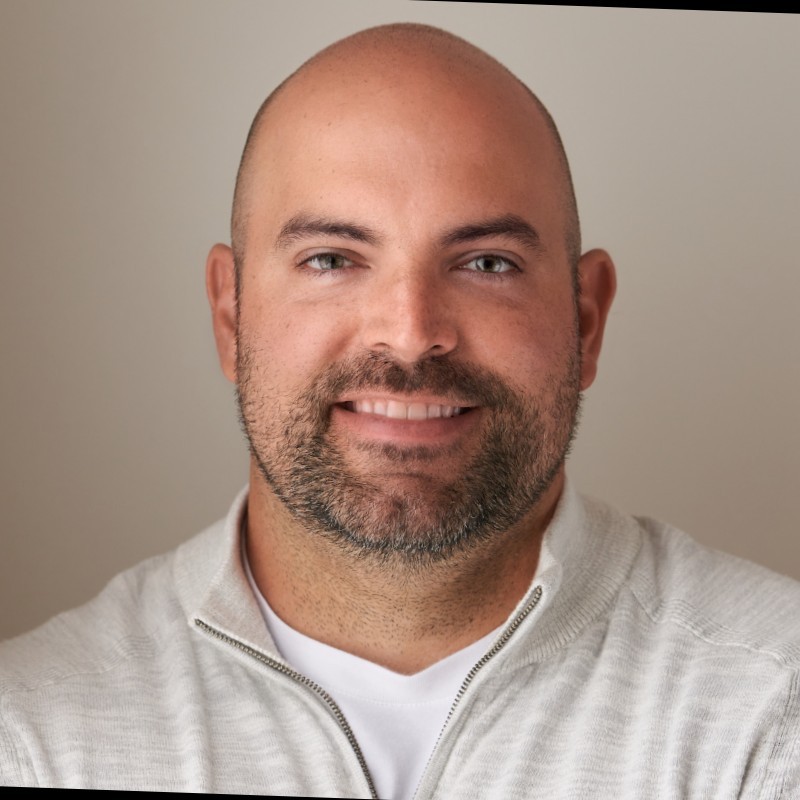
Master Native Stripe Integration for mobile apps with this complete guide, covering setup, security, and customization for a seamless payment experience.
Building a mobile app and want a smooth, in-app payment experience? Forget redirecting users to external websites. Native Stripe Integration lets your customers complete purchases directly within your app, creating a more professional and user-friendly checkout flow. This article breaks down everything you need to know about Native Stripe Integration with React Native, from setting up your Stripe account to optimizing the payment process for a seamless user experience. We'll cover key features, security best practices, and troubleshooting tips to help you build a secure and efficient payment system. Whether you're building an e-commerce app, a subscription service, or anything in between, this guide will equip you with the knowledge to implement Native Stripe Integration effectively.
Native Stripe integration seamlessly adds Stripe's payment processing features directly into your mobile app. Instead of redirecting customers to an external website, they complete purchases within your app, creating a smoother, more professional user experience. Think of popular apps like Instacart or Lyft—they use native integrations for payments. This approach lets developers build a checkout flow that feels like a natural part of the app.
A key advantage of using Stripe is enhanced security. Stripe handles sensitive card data directly, reducing your PCI compliance burden and simplifying development so you can focus on other aspects of your app. Plus, a streamlined checkout process generally leads to higher conversion rates. No one wants to leave an app mid-purchase, so keeping users within your app can significantly improve sales. Stripe offers resources on how to best manage customer card information securely.
Stripe's Native SDKs provide tools like the Payment Sheet, a pre-built component that handles collecting payment information and confirming transactions. This simplifies integration and ensures a consistent user experience across different platforms. The React Native SDK, for example, makes it easy to add secure payment processing to both Android and iOS apps. It handles the complexities of secure data transmission and PCI compliance, freeing you to focus on building a great app. You can find more information on Stripe's various SDKs in their documentation.
Before diving into the code, let's set up everything you'll need for a smooth integration. Think of this as prepping your ingredients before you start cooking.
First things first, you'll need a Stripe account. If you don't already have one, head over to their website and sign up. It's straightforward and shouldn't take long. Once you're in, locate your API keys. These are crucial for connecting your app to Stripe and securely processing payments. Keep them confidential—treat them like your secret recipe! You'll use your publishable key to initialize Stripe in your React Native app, either through the StripeProvider
component or the initStripe
initialization method. This sets the stage for secure communication between your app and Stripe's services.
With your Stripe account ready, the next step is installing the required libraries. Think of these as your essential kitchen tools. You can add the Stripe React Native SDK to your project using your preferred package manager:
npm install @stripe/stripe-react-native
yarn add @stripe/stripe-react-native
expo install @stripe/stripe-react-native
(and remember to configure your app.json
accordingly)This SDK is purpose-built for handling payments in mobile apps, ensuring sensitive data is managed securely and you maintain PCI compliance. It's like having a sous chef specializing in security and compliance, making your life much easier.
This section provides a practical walkthrough for integrating Stripe into your React Native application. We'll cover setting up the SDK, building payment components, implementing the Payment Sheet, and handling user authentication.
First, install the Stripe React Native SDK using either npm or yarn. This equips your app with the tools to communicate with Stripe's services. It provides pre-built UI elements and functions, simplifying the integration. After installation, link the native libraries. This step is essential for proper communication between the SDK and the native platform.
Next, create the components that will handle payments within your app. While you can build custom payment forms, Stripe's Payment Sheet offers a streamlined, pre-built solution. This single, customizable screen collects payment details and confirms the payment securely within your app, reducing development time. You'll use this component to interact with the Stripe SDK.
To display and manage the Payment Sheet, use the initPaymentSheet
and presentPaymentSheet
functions from the Stripe React Native SDK. The initPaymentSheet
function configures the Payment Sheet with parameters like your payment intent client secret and any desired customization options. After initialization, call presentPaymentSheet
to display the Payment Sheet to the user. This approach simplifies the payment process, providing a user-friendly interface for entering payment information.
When processing payments, users might need to authenticate through 3D Secure or other verification methods. This often involves redirecting them to an external app, like their banking app. To ensure a smooth return to your app after authentication, correctly setting up a return URL is crucial, especially for iOS. This return URL directs the user back to your app after completing authentication, preventing them from getting lost during the redirect.
Security is paramount when dealing with financial transactions. Let's explore some key practices to ensure your Stripe integration is as secure as possible.
Before launching your app, always test your integration using Stripe's test API keys. This lets you simulate transactions without processing real payments. Once you're ready to go live, switch to your live keys. Remember, handling sensitive card information directly puts the onus on you to maintain PCI compliance, which can be complex. Stripe strongly recommends using their payment methods, like the Payment Sheet or Payment Intents API, to minimize your PCI burden. These methods tokenize sensitive data, meaning your app never directly handles it. Always use SSL encryption (HTTPS) for all communication between your app and Stripe's servers. This encrypts data in transit, protecting it from eavesdropping.
The Payment Card Industry Data Security Standard (PCI DSS) is a set of security standards designed to protect cardholder data. When you use Stripe, they handle the heavy lifting of PCI compliance as a Level 1 certified service provider. However, understanding your responsibilities is still crucial. By leveraging Stripe's pre-built tools and APIs, like their client-side encryption and tokenization features, you significantly reduce your PCI scope. This means less paperwork and fewer security headaches. If you do need to handle sensitive card data directly (for example, if you're using a custom payment form), you'll need to familiarize yourself with the requirements of PCI DSS and ensure your app meets them.
Stripe's APIs are designed with security in mind, but it's important to handle errors gracefully and protect user data on your end. Implement robust error handling in your app to catch any issues during payment processing. Display user-friendly error messages and log errors on the backend for debugging. Never store sensitive card data directly within your app or on your servers unless absolutely necessary. If you must store card data, follow Stripe's best practices for secure storage and encryption. Remember, security is a continuous process, so stay informed about security best practices and update your integration regularly to address any potential vulnerabilities.
After you’ve integrated Stripe, the next step is managing the payment process itself. This involves handling transactions, refunds, and staying on top of payment events. Let's break down how to do this effectively.
To process transactions smoothly, use Stripe’s PaymentIntent
object. This represents your intent to collect a payment and manages the entire lifecycle. Think of it as the conductor of your transaction orchestra. You'll also want to create Customer
objects. Storing customer payment information makes repeat purchases easier and improves the user experience. Returning customers won't have to re-enter their details every time, making checkout faster and more convenient.
Handling refunds is equally important. A clear and efficient refund process builds trust with your customers. Stripe provides APIs to process refunds programmatically, allowing you to manage refunds directly within your app. For more details on implementing these objects and managing refunds, check out the Stripe documentation.
Webhooks are your secret weapon for managing events after a payment is initiated. They act like real-time messengers, notifying your app about changes in payment status. This is much more reliable than relying on client-side callbacks. Set up webhooks to listen for key events like payment_intent.succeeded
, payment_intent.processing
, and payment_intent.payment_failed
. This lets you automate actions like order fulfillment or sending confirmation emails.
Responding promptly to payment confirmations and failures is crucial for a positive user experience. When a payment is successful, immediately notify the user and trigger any necessary backend processes, such as order fulfillment. If a payment fails, handle it gracefully. Provide clear error messages to the user and offer solutions, like retrying the payment or using a different payment method. This proactive approach keeps your customers informed and minimizes frustration. You can find a comprehensive guide to setting up webhooks and handling payment events in the Stripe documentation.
Once you’ve integrated Stripe, you can customize the payment experience to match your app’s branding and target audience. This improves user experience and encourages conversions.
Think through the steps a user takes to complete a purchase. The Stripe React Native SDK provides pre-built UI elements that simplify creating a smooth payment flow. These pre-built components handle complex tasks behind the scenes, so you can focus on making the process intuitive and user-friendly.
Offering the right payment options is crucial for a positive user experience. Stripe’s flexibility lets you support various payment methods, from traditional credit cards to bank redirects and debits. You can manage these options through the Stripe dashboard or manually specify them within your app. Stripe automatically prioritizes payment methods based on the user’s currency and location, creating a more intuitive checkout. For more details, check out Stripe’s documentation on accepting payments.
While Stripe’s pre-built UI components are helpful, sometimes you need a more tailored look. You can use PaymentSheet to seamlessly integrate payments into your custom UI. This gives you complete control over the payment experience while still leveraging Stripe’s powerful backend. The @stripe/stripe-react-native
package provides native-looking payment screens for both Android and iOS, ensuring a consistent and polished experience for everyone.
Thoroughly testing your Stripe integration before launch is crucial for a smooth user experience. Thankfully, Stripe offers robust tools to make this process straightforward.
Stripe provides comprehensive testing tools that let you simulate various payment scenarios without using real money. This is essential for identifying and fixing issues early on. You can use test card numbers and create test PaymentIntents, ensuring your integration handles different situations correctly. Think of it as a dress rehearsal for your payment system. This allows you to confidently handle everything from successful transactions to declined cards.
Using Stripe's test card numbers, you can mimic a range of payment outcomes. Simulate successful payments to confirm your basic flow is working. Then, test scenarios like authentication required or declined cards to ensure your app responds gracefully. This testing process helps you prepare for real-world situations and avoid unexpected issues down the line. By testing these edge cases, you'll create a more resilient and user-friendly payment experience.
Even with careful planning, integration hiccups can happen. Common challenges include handling asynchronous events, using API keys correctly, and managing webhooks for post-payment events. Refer to the Stripe documentation for troubleshooting tips and best practices to resolve these issues effectively. It's a valuable resource that can save you time and frustration. Don't hesitate to consult it when you encounter a roadblock. Additionally, exploring community forums can provide insights and solutions from other developers who have faced similar challenges.
A smooth and efficient payment process is crucial for a positive user experience. This section covers key strategies to optimize performance and ensure your users enjoy a seamless checkout.
No one likes to wait, especially when making a purchase. Integrating Stripe into your application can be straightforward, but ensuring efficient payment processing requires attention to detail. Minimize latency by optimizing your network requests and leveraging Stripe's pre-built components. One common pitfall is unnecessary data processing during the payment flow. Streamline this process to reduce delays and keep the user experience snappy. For more insights on integrating Stripe smoothly, check out this helpful article on common Stripe integration challenges.
Keep users informed throughout the payment process with real-time feedback. Display clear loading indicators while processing transactions and provide immediate confirmation upon successful payment. Stripe's documentation offers guidance on handling post-payment events (webhooks), which are essential for providing real-time updates. Utilize these webhooks to inform the user about the transaction status. This transparency builds trust and reduces user anxiety.
Even with the best planning, errors can occur. A robust payment integration should include comprehensive error handling. Implement clear error messages that guide users toward a solution, whether it's retrying the payment or contacting support. Consider incorporating retry mechanisms to handle temporary network issues gracefully. Addressing compatibility issues and security concerns, along with performance optimization, are key to creating a reliable application. This article on Stripe developer challenges provides further insights into building a robust and reliable payment experience.
Integrating Stripe into your mobile app is generally straightforward, thanks to their comprehensive documentation and active community support. Stripe offers a variety of SDKs to simplify payment processing across different programming languages and platforms, making it easier to build a payment solution tailored to your needs. For detailed instructions and best practices, refer to the official Stripe documentation, which is regularly updated. Beyond the official resources, engaging with the Stripe community in forums can offer valuable insights and solutions.
Staying informed about new Stripe features and security best practices is essential for a secure and efficient payment integration. When passing card information directly to Stripe’s API, ensure you maintain PCI compliance. Handling sensitive card data directly increases the complexity of these requirements. Processing payments through Stripe minimizes your compliance burden since you avoid directly handling sensitive data. Regularly review Stripe’s security guides and compliance resources to stay on top of the necessary measures to protect customer data. Stripe is constantly evolving, so keeping current with their latest updates ensures your integration remains secure, optimized, and leverages the newest functionalities.
Why should I use a native Stripe integration instead of a web-based checkout? A native integration keeps users within your app, creating a smoother experience that generally leads to higher conversion rates. It also allows for deeper customization and branding, making the checkout process feel like a natural part of your app.
What are the security advantages of using Stripe's native SDKs? Stripe handles sensitive card data directly, reducing your PCI compliance burden. Features like the Payment Sheet and pre-built UI components further enhance security by minimizing the amount of sensitive data your app needs to handle.
What's the difference between Stripe's test and live API keys? Test keys allow you to simulate transactions without processing real payments, crucial for testing your integration before going live. Live keys are used for processing real transactions once your integration is thoroughly tested and ready for production.
What are webhooks and why are they important for managing payments? Webhooks are real-time notifications from Stripe that inform your app about changes in payment status. They're more reliable than client-side callbacks and allow you to automate actions like order fulfillment or sending confirmation emails.
How can I customize the Stripe payment experience in my app? You can customize the payment flow, localize currency and payment methods, and even implement custom UI elements while still leveraging Stripe's secure backend. This allows you to create a branded payment experience that seamlessly integrates with your app's design.
Former Root, EVP of Finance/Data at multiple FinTech startups
Jason Kyle Berwanger: An accomplished two-time entrepreneur, polyglot in finance, data & tech with 15 years of expertise. Builder, practitioner, leader—pioneering multiple ERP implementations and data solutions. Catalyst behind a 6% gross margin improvement with a sub-90-day IPO at Root insurance, powered by his vision & platform. Having held virtually every role from accountant to finance systems to finance exec, he brings a rare and noteworthy perspective in rethinking the finance tooling landscape.